λμ΄λ: Medium
ν€μλ: Hash Table
π² λ¬Έμ
X-Y νλ©΄μμ μ λ€μ λ°°μ΄ pointsκ° μ£Όμ΄μ‘μ΅λλ€. μ¬κΈ°μ points[i] = [xi, yi]μ
λλ€.
μ΄λ¬ν μ λ€λ‘ νμ±λ XμΆκ³Ό YμΆμ ννν μ§μ¬κ°νμ μ΅μ λ©΄μ μ λ°νν©λλ€. λ§μ½ κ·Έλ¬ν μ§μ¬κ°νμ΄ μλ€λ©΄, 0μ λ°νν©λλ€.
λ¬Έμ μλ¬Έ: https://leetcode.com/problems/minimum-area-rectangle/description/
Example 1:
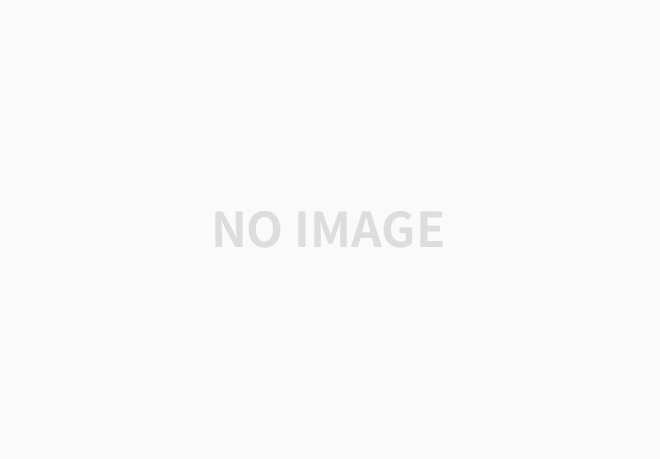
Input: points = [[1,1],[1,3],[3,1],[3,3],[2,2]]
Output: 4
π² λ¬Έμ νμ΄
λ§λ€ μ μλ μ¬κ°νμ νμνλ©΄μ μ¬κ°νλ€ μ€ μ΅μ λ©΄μ κ°μ ꡬνκ³ μνλ€.
μ΄λ₯Ό μν΄μ λ κ°μ μ μ΄ μ£Όμ΄μ‘μ λ μ΄ λ μ μΈμ μ¬κ°νμ λ§λ€ μ μλ μ λ€μ΄ μ‘΄μ¬νλ μ§ O(1) μΌλ‘ νμνλ ννλ‘ μ¬κ°ν λ©΄μ μ ꡬν μ μλ€.
class Solution {
public:
int minAreaRect(vector<vector<int>>& points) {
unordered_map<int, set<int>> hash;
for(auto point : points){
auto x = point[0], y = point[1];
hash[x].insert(y);
}
int MAX = 16 * pow(10, 8);
int ans = MAX;
for(auto i=0; i < points.size()-1; ++i){
for(auto j=i; j < points.size(); ++j){
auto x1 = points[i][0], y1 = points[i][1];
auto x2 = points[j][0], y2 = points[j][1];
if(x1 == x2) continue;
if(y1 == y2) continue;
// check rectangle
if(hash[x1].find(y2) != hash[x1].end() && hash[x2].find(y1) != hash[x2].end())
ans = min(ans, abs(x1-x2) * abs(y1-y2));
}
}
if(ans == MAX)
ans = 0;
return ans;
}
};
ν΄λΉ μ±λμμ Leet Code ν΄μ€ν΄ λμ κ²λ€μ΄ μλλ° κ³Όμ μ΄ μ²΄κ³μ μ΄κ³ μ’μ κ² κ°λ€ :)
https://youtu.be/xvuuENPhEH4?feature=shared
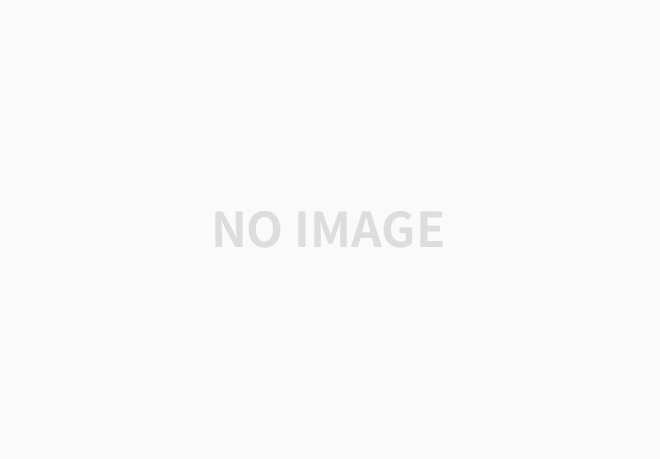
'IN DEPTH CAKE > Supercoder' μΉ΄ν κ³ λ¦¬μ λ€λ₯Έ κΈ
[c++/LeetCode-DP] 746. Min Cost Climbing Stairs (1) | 2024.05.06 |
---|---|
[c++/LeetCode-DP] 70. Climbing Stairs (1) | 2024.05.06 |
[c++/LeetCode-Hash Table] 692. Top K Frequent Words (1) | 2024.05.05 |
[c++/LeetCode-Hash Table] 387. First Unique Character in a String (1) | 2024.05.05 |
[c++/LeetCode-Hash Table] 1. Two Sum (1) | 2024.05.05 |